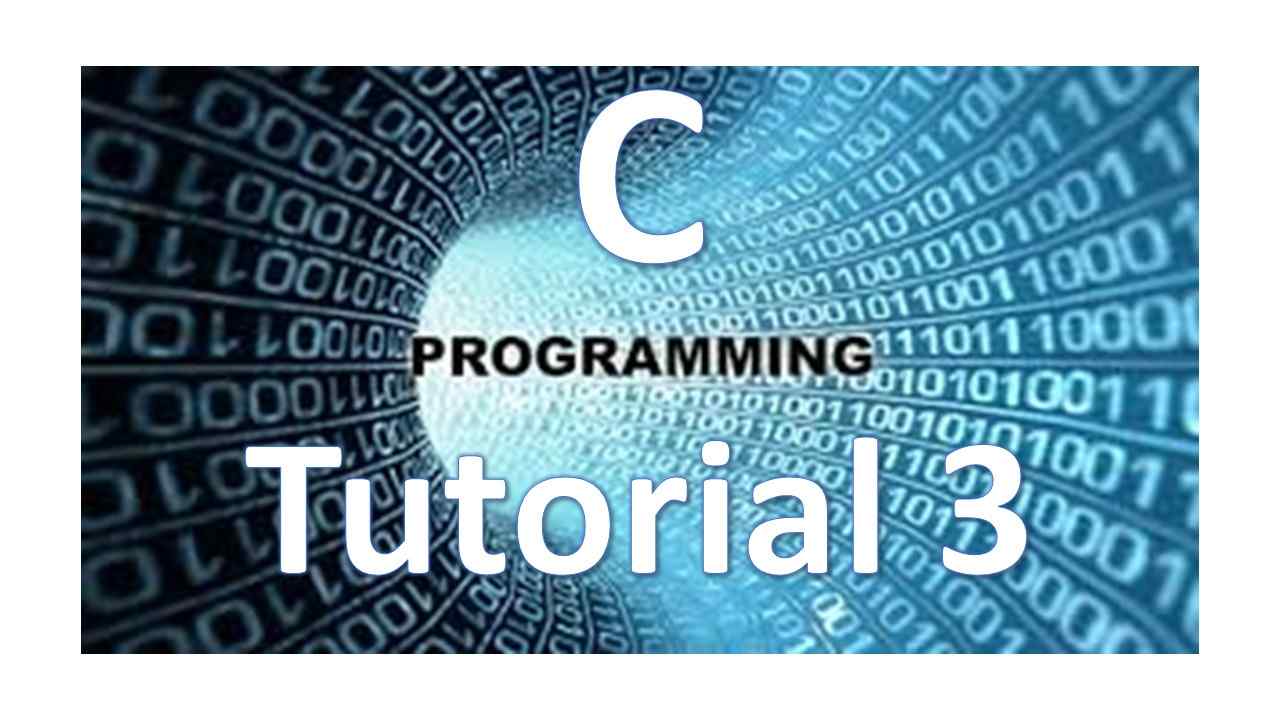
Welcome to C Programming Tutorial three!
In the last C programming tutorial, we talked about what a variable is and went over some common variable types, explaining what they’re used for and what their limitations are.
We’re still learning the basics of C programming, so this time we’re going to talk about arithmetic and logic.
Logic and arithmetic are one of the foundations of any programming language.
Some may think the principles and rules on this are obvious and may be tempted to skip this tutorial. I urge you not to skip, especially if you’re new to C programming. While many things about arithmetic and logic may seem obvious and boring, there are some caveats and gotchas. Even if you’re a C programming aficionado, this tutorial can serve as a good review of the basics.
Sometimes, gaining more complex knowledge has the tendency to push simple things into the dusty corners of our brains, making us forget the basics and over-complicate things.
Let’s do this thing.
C Programming Tutorial 3: Arithmetic and Logic
Arithmetic in C
Almost all the programs you’ll write will perform some sort of arithmetic operations.
Most third graders know what addition, subtraction, multiplication and division are, so I’ll spare you the gory details on how those work. And this isn’t a lesson on math anyway.
I will, however, give the symbols in C for each of the arithmetical operators. Also, since some readers may have forgotten this (or are unfamiliar with it) we’ll quickly go over the order of operations.
The symbols for addition and subtraction in C/C++ are the same ones you’re familiar with.
To multiply in C, you use an asterisk (*). For example, the statement
c = b *27;
multiplies b by 27 and then assigns that number to variable c. We’ll talk more about the assignment operator in a minute.
To divide in C, you use the forward slash (/). For example, the statement
c = b / 27;
divides b by 27 and then assigns that number to variable c.
Here comes the first caveat of arithmetic in C that will make reading this post worthwhile: integer division yields an integer quotient. Understanding this will save you hours of frustration trying to track down hard-to-find logical bugs.
Ever dreamed of being Iron Man, having the ability to build anything, anytime? Try Academy for Arduino!
In other words, any fraction resulting from integer division is discarded. This process is called truncation.
To illustrate, consider the arithmetic problem 5 divided by 4. A calculator will give you an answer of 1.25. The C language will give you an answer of 1, dropping the 0.25. Similarly, dividing 7 by 4 will give an answer of 1 rather than 1.75.
The float variable type will get you around this little gotcha. So, if I want to divide 5 by 4 and get a true answer, I would write:
c = 5.0 / 4.0;
and get an answer of 1.25 for the variable c.
When you mix integers with floating point numbers, the answers come out the same as floating point arithmetic. The computer is not really capable of dividing a floating point type by an integer type, so the compiler converts both operands to a single type. In this case, the integer converts to floating point before the division takes place. For example, writing:
c = 5.0 / 4;
still gives an answer or 1.25 rather than truncating and giving you an answer of 1. The nice compiler converts the integer 4 to a float of 4.0 for you without bugging you.
Order of Operations in C Programming
The order of operations in C is the same as the order of operations in arithmetic and algebra – with one little exception.
Some of us may remember math teachers giving us a handy little mnemonic for remembering the order of operations.
The mnemonic is Please Excuse My Dear Aunt Sally (PEMDAS). At least that’s the one I was taught.
Figure 1 is a graphical representation of this memory aid.
Figure 1: graphical representation of the order of operations mnemonic.
Like algebra, any term in parentheses comes first, regardless off the operation. Then things get sticky.
Here’s the second caveat of arithmetic in C that will make reading this post worthwhile: C does not have an exponential operator (that’s the little exception from earlier). The standard C math library, however, provides the pow() function for that purpose. For example, pow(3, 2) returns 3 raised to the power of 2.
So, writing something like
c = b^2; // tries to set variable c to b squared and fails – big time.
will NOT work and will result in a syntax error.
Another caveat that many of us are already familiar with is that multiplication and division in algebra (and C programming) have the same level of precedence. The same is true for addition and subtraction, though multiplication and division have a higher precedence than adding and subtracting.
Using the order of operations, an expression like
c = 2 * 3 + 8 / 2; // demonstrates order of operations.
sets variable c equal to 10.
If we were to instead write:
c = 2 * (3 + 8) / 2; // also demonstrates order of operations.
we would be setting variable c equal to 11 because the junk in the parentheses has a higher precedence and evaluates first. It does not matter whether we choose to do the multiplication or the division first, in either case, the answers remain the same.
There is yet another operator holding equal ranking with multiplication and division in C. Some of you may not have even heard of it.
Modulo Division in C Programming
If you’re a programming veteran, you’re likely familiar with modulo division. If you’re new, you may not have heard of modulo (a.k.a. modulus) division and it may seem a bit weird. Therefore, I’m giving it its own section because these posts assume you’re new to C programming.
When you were first learning long division in grade school, you may have been using modulo division (sort of) without even knowing it.
For example, you may remember doing a problem like 7 divided by 4. The answer is 1 with a remainder of 3. Perhaps later you learned how to get the more exact answer of 1.75 using long division (both answers are technically correct).
The modulus operator is used in integer arithmetic. It gives the remainder that results when the integer to its left is divided by the integer to its right. For example, 13 % 5 (read as 13 modulo 5) has the value 3, because 5 goes into 13 twice, with a remainder of 3. Don’t try to use this operator with floating-point numbers. It won’t work. Let’s look at some quick examples.
c = 13 % 5; // sets variable c equal to 3 because 5 goes into 13 twice with a remainder of 3. c = 3.14 % 1.1; // Fail! The modulo operator only works on integers.
If you’re a programming noob, right now, you may be thinking something like why the hell would I want to throw away the quotient and keep the remainder?
Trust me, the modulo operator is like that friend you don’t see every day, but when you call them for help they’re always there with bells on. You may not use it in every program, but you will eventually use it and it will make your life easier.
A quick example of a practical use for the modulo operator would be to determine if one number is a multiple of another. If so, the remainder using modulo division on the two numbers will be zero. There are many other uses.
One final note: the modulo operator has the same level pf precedence as multiplication and division. Perhaps we can change our mnemonic to Please Excuse My Magnificent Dear Aunt Sally (PEMMDAS).
Or not.
C Programming Tutorial 3: Assignment Operator vs Equal Sign
It’s time that we bust this wide open. This is something that confuses many programmers, especially new ones.
In C, the equal sign does not mean “equals.” Rather, it is a value-assigning operator or assignment operator.
The statement
year = 2018;
assigns the value to 2018 to the variable year.
The item to the left of the = sign is the name of a variable, and the item on the right is the value assigned to the variable. Don’t think of the line as saying, “year equals 2018.” Instead, read it as “assign the value 2018 to the variable year.”
Most operators evaluate from left to right. The assignment operator evaluates from right to left.
The equality operator is two equal signs or ==. This operator should be read as “is equal to.”
You may be wondering when to use the equality operator. This snippet of code gives an example:
if (a == b) // Interesting code goes here
This statement says “if a equals b, then do something…”
We’ll talk more about if statements and other control structures in another C programming tutorial.
Confusing the assignment operator (=) with the equality operator (==) can result in logic errors that may be hard to find and debug.
Other C Equality Operators & Relational Operators
Now that we know what the assignment operator and the equality operator are (and the difference between them) we need to talk about a few other handy operators you’ll often use.
There are actually two equality operators in C. The second one is the “not equal” operator which looks like !=. The code snippet below suggests some uses for it.
If (a != B) // Interesting code goes here
This statement says “if a is not equal to b, then do something…”
Most of us are familiar with the other relational operators (assuming we’ve graduated second grade).
There is the greater than operator (>), the less than operator (<), the greater than or equal to (>=) and the less than or equal to (<=) operators.
They mean exactly what you think they mean, so again I won’t go into gory details about them, but know that they come in handy for things like if statements and other control structures.
We’ll summarize all these operators in a nifty table for you near the end of the post, but first there’s one more type of operator we need to talk about.
Two Important C Logical Operators
To understand how C logical operators work, an understanding of basic gates and digital logic is helpful. If you’re rusty with that sort of thing, read A Tutorial on Logic Gates first, then come back here. You can skip most of it if you want and just read the first part dealing with AND and OR for the purposes of this discussion.
The two logical operators we’ll concern ourselves with here are the logical AND (&&) and logical OR (||).
You’ll often find yourself using one or both in your programming. To get an idea of how to use them, consider the code snippets below.
if (height >= 48 && age >= 6) printf (“You can get on this ride.”); /* if height is greater than or equal to 48” and age is at least 6. This carny is being safe and cautious. */ if (height >= 48 || age >= 6) printf (“You can get on this ride.”); /* if height is greater than or equal to 48” or your age is at least 6. This carny might get himself in trouble. */
I’m sure you can think of a hundred other instances in which these operators would come in handy.
C Programming Tutorial 3 Wrap-up
As promised, before we wrap up, figure 2 below depicts a summary of all the operators we talked about in C programming tutorial 3. Bookmark this page.
Figure 2: common C programming operators, their symbols, and associativity.
As a bonus, I’m throwing in figure 3, which shows the level of precedence of each operator. The top items have a higher precedence than the bottom ones. Operators with the same level of precedence are in the same row.
Figure 3: common C programming operator precedence.
Next time, we’ll get into those mysterious if statements and control structures that keep coming up. Keep reading the C Programming Tutorials. Next thing you know, you’ll be a C whiz.
Comment and tell us about your latest C program. What does it do? Was it difficult to write? If so, what made it hard? We’d love to hear about it!
Ever dreamed of being Iron Man, having the ability to build anything, anytime? Try Academy for Arduino!

Electronics Tips & Tutorials Sent Directly to Your Inbox

Submit your email & you'll get:
- Exclusive content that I don't put on the blog
- The checklist 10 mistakes all electronics enthusiasts make (& how to avoid them)
- And more!
a vEry interesting content;a lot of appreciation.with consideration
Better and more sensible than anything programming that i ever tried
I haven’t begun to code anything yet. I mean I wrote hello world but I am just reading. I don’t think I could even comprehend the sentence where I to write a line of code.